This post will discuss utilizing Python scripts to automate sending emails. For this example, we will be simulating sending an email based off of a triggered alert.
First, we will need to write and send an email with the template for the alert.
The all capitalized words will be replaced with the information pertinent to the email we want to send. The next step is to highlight the fist line of the email body and inspect the element to show us the HTML data. We can copy the HTML element and paste it into a new file. I will name it message.html
.
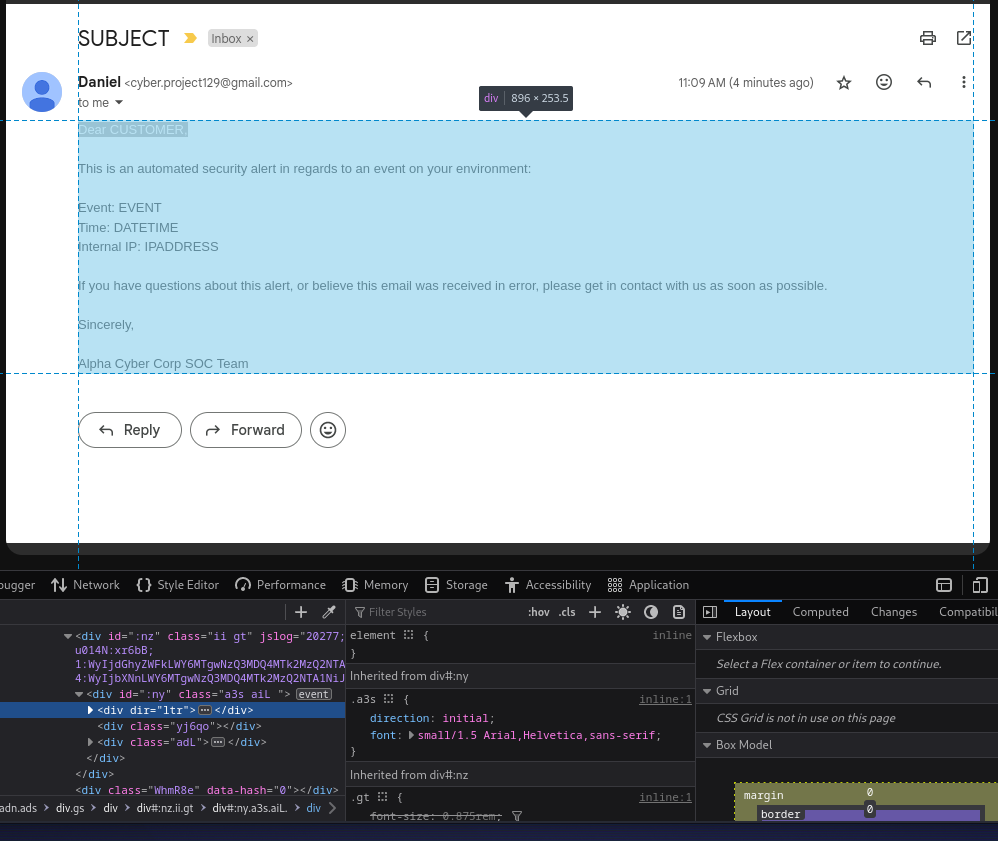
From here, we can check to see if the copied element gives us what we want by opening a browser and following the file pathway.
Looks like we are good.
Now we need a Python script:
After we run this script, it creates the sent_email.html
file. We can follow the file path in a browser again to see what the email will look like:
As we can see, the all caps placeholder words have been replaced with the necessary data from the variables in the script.
Now that we know the script is working, we need to modify it to send as an actual email, as well as have an email account set up to do the sending. We will tackle the second part first by going into the security settings of a Gmail account I created, enabling MFA, and going to app passwords. Enter the necessary info and we get an app password. The password is only shown once, so we need to write it down to use in the script. In a real world use case, we would want to use a secrets manager for better security, but this will suffice for this tutorial.
Now that we have the email account set up, we need a method to send an email via Python. For this tutorial we will use yagmail. Perform a pip3 install if needed, and then edit the script to add the necessary information.
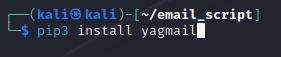
Here is the new script. It is the same base script as before with some added elements. We have to import the yagmail package at the top in addition to the datetime package. I also commented out the the two lines that create and write to the sent_email.html
file because we don’t need to write the file every time.
In the variable section, I added a few variable definitions to incorporate into the yagmail section at the bottom.
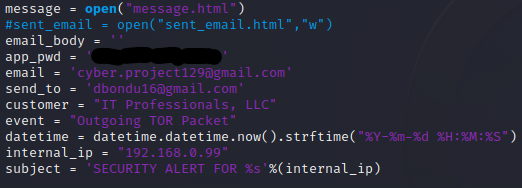
In this last section, the email_body += line
will write each line into the email body. The last part is the yagmail block that takes everything and puts it together into the email and sends it out.
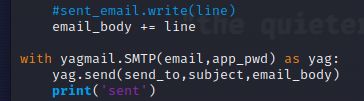
Now when we run the script, we want to see “sent” print out in the terminal:
And here is the email:
Looks like everything is working correctly. In a real world production setting this script would be one part of a larger process to send out security alert information.